JsRender jot down
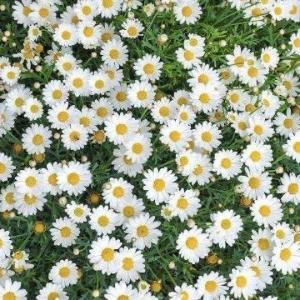
JsRender is a lightweight, powerful and highly extensible templating engine.
download:https://github.com/borismoore/jsrender
Define a template
From a string:
var tmpl = $.templates("Name: {{:name}}");
From a template declared as markup in a script block:
<script id="myTemplate" type="text/x-jsrender">
Name: {{:name}}
</script>
then, somewhere in your script:
var tmpl = $.templates("#myTemplate");
From an .html file containing the template markup:
var tmpl = $.templates("./templates/myTemplate.html");
Render a template
tmpl.render(object)
(or shortcut form: tmpl(object)
) renders the template with the object as data context.
var tmpl = $.templates(" Name: {{:name}}<br/> ");
var person = {name: "Jim"};
// Render template for person object
var html = tmpl.render(person); // ready for insertion, e.g $("#result").html(html);
// result: "Name: Jim<br/> "
tmpl.render(array)
(or tmpl(array)
) renders the template once for each item in the array.
var people = [{name: "Jim"}, {name: "Pedro"}];
// Render template for people array
var html = tmpl.render(people); // ready for insertion...
// result: "Name: Jim<br/> Name: Pedro<br/> "
Register a named template - and render it
// Register named template - "myTmpl1
$.templates("myTmpl1", "Name: {{:name}}<br/> ");
var person = {name: "Jim"};
// Render named template
var html = $.templates.myTmpl1(person);
// Alternative syntax: var html = $.render.myTmpl1(person);
// result: "Name: Jim<br/> "
Template tags
Template tag syntax
-
All tags other than
{{: ...}}
{{> ...}}
{{* ...}}
{{!-- --}}
behave as block tags -
Block tags can have content, unless they use the self-closing syntax:
- Block tag - with content:
{{someTag ...}} content {{/someTag}}
- Self-closing tag - no content (empty):
{{someTag .../}}
- Block tag - with content:
-
A particular case of self-closing syntax is when any block tag uses the named parameter
tmpl=...
to reference an external template, which then replaces what would have been the block content:- Self-closing block tag referencing an external template:
{{someTag ... tmpl=.../}}
- Self-closing block tag referencing an external template:
-
Tags can take both unnamed arguments and named parameters:
{{someTag argument1 param1=...}} content {{/someTag}}
- an example of a named parameter is the
tmpl=...
parameter mentioned above - arguments and named parameters can be assigned values from simple data-paths such as
address.street
or from richer expressions such asproduct.quantity * 3.1 / 4.5
, orname.toUpperCase()
Built-in tags
{{: ...}} (Evaluate)
{{: pathOrExpr}}
inserts the value of the path or expression.
var data = {address: {street: "Main Street"} };
var tmpl = $.templates("<b>Street:</b> {{:address.street}}");
var html = tmpl.render(data);
// result: "<b>Street:</b> Main Street"
{{> ...}} (HTML-encode)
{{> pathOrExpr}}
inserts the HTML-encoded value of the path or expression.
var data = {condition: "a < b"};
var tmpl = $.templates("<b>Formula:</b> {{>condition}}");
var html = tmpl.render(data);
// result: "<b>Formula:</b> a < b"
{{include ...}} (Template composition - partials)
{{include pathOrExpr}}...{{/include}}
evaluates the block content against a specified/modified data context.
{{include ... tmpl=.../}}
evaluates the specified template against an (optionally modified) context, and inserts the result. (Template composition).
var data = {name: "Jim", address: {street: "Main Street"} };
// Register two named templates
$.templates({
streetTmpl: "<i>{{:street}}</i>",
addressTmpl: "{{:name}}'s address is {{include address tmpl='streetTmpl'/}}."
});
// Render outer template
var html = $.templates.addressTmpl(data);
// result: "Jim's address is <i>Main Street</i>"
{{for ...}} (Template composition, with iteration over arrays)
{{for pathOrExpr}}...{{/for}}
evaluates the block content against a specified data context. If the new data context is an array, it iterates over the array, renders the block content with each data item as context, and concatenates the result.
{{for pathOrExpr tmpl=.../}}
evaluates the specified template against a data context. If the new data context is an array, it iterates over the array, renders the template with each data item as context, and concatenates the result.
<script id="peopleTmpl" type="text/x-jsrender">
<ul>{{for people}}
<li>Name: {{:name}}</li>
{{/for}}</ul>
</script>
var data = {people: [{name: "Jim"}, {name: "Pedro"}] };
var tmpl = $.templates("#peopleTmpl");
var html = tmpl.render(data);
// result: "<ul> <li>Name: Jim</li> <li>Name: Pedro</li> </ul>"
{{props ...}} (Iteration over properties of an object)
{{props pathOrExpr}}...{{/prop}}
or {{props pathOrExpr tmpl=.../}}
iterates over the properties of the object returned by the path or expression, and renders the content/template once for each property - using as data context: {key: propertyName, prop: propertyValue}
.
<script id="personTmpl" type="text/x-jsrender">
<ul>{{props person}}
<li>{{:key}}: {{:prop}}</li>
{{/props}}</ul>
</script>
var data = {person: {first: "Jim", last: "Varsov"} };
var tmpl = $.templates("#personTmpl");
var html = tmpl.render(data);
// result: "<ul> <li>first: Jim</li> <li>last: Varsov</li> </ul>"
{{if ...}} (Conditional inclusion)
{{if pathOrExpr}}...{{/if}}
or {{if pathOrExpr tmpl=.../}}
renders the content/template only if the evaluated path or expression is 'truthy'.
{{if pathOrExpr}}...{{else pathOrExpr2}}...{{else}}...{{/if}}
behaves as 'if' - 'else if' - 'else' and renders each block based on the conditions.
<script id="personTmpl" type="text/x-jsrender">
{{if nickname}}
Nickname: {{:nickname}}
{{else name}}
Name: {{:name}}
{{else}}
No name provided
{{/if}}
</script>
var data = {nickname: "Jim", name: "James"};
var tmpl = $.templates("#personTmpl");
var html = tmpl.render(data);
// result: "Nickname: Jim"
Helpers
Here is a simple example. Two helpers - a function, and a string:
var myHelpers = {
upper: function(val) { return val.toUpperCase(); },
title: "Sir"
};
Access the helpers using the ~myhelper
syntax:
var tmpl = $.templates("{{:~title}} {{:first}} {{:~upper(last)}}");
We can pass the helpers in with the render()
method
var data = {first: "Jim", last: "Varsov"};
var html = tmpl.render(data, myHelpers);
// result: "Sir Jim VARSOV"
Or we can register helpers globally:
$.views.helpers(myHelpers);
var data = {first: "Jim", last: "Varsov"};
var html = tmpl.render(data);
// result: "Sir Jim VARSOV"
Converters
Converters are used with the {{:...}}
tag, using the syntax {{mycvtr: ...}}}
.
Example - an upper converter, to convert to upper case:
$.views.converters("upper", function(val) { return val.toUpperCase(); });
var tmpl = $.templates("{{:first}} {{upper:last}}");
var data = {first: "Jim", last: "Varsov"};
var html = tmpl.render(data);
// result: "Jim VARSOV"
Logic and expressions
JsRender supports rich expressions and logic, but at the same time encapsulates templates to prevent random access to globals. If you want to provide access to global variables within a template, you have to pass them in as data or as helpers.
You can assign rich expressions to any template arguments or parameters, as in:
{{:person.nickname ? "Nickname: " + person.nickname : "(has no nickname)"}}
or
{{if ~limits.maxVal > (product.price*100 - discount)/rate}}
...
{{else ~limits.minVal < product.price}}
...
{{else}}
...
{{/if}}
本文链接 https://www.mangoxo.com/blog/A3DObyxV 版权所有,转载请保留地址链接,感谢!
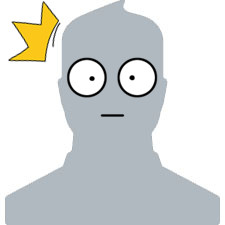